mirror of
https://codeberg.org/Amm0ni4/bypass-all-shortlinks-debloated.git
synced 2024-12-28 07:53:01 +05:00
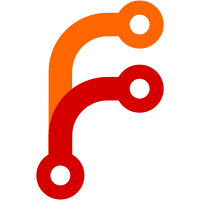
add recaptcha/api2 to match, so they are auto open when that option is enabled. Also added domain shrinkme.us which has one of those captchas
94 lines
2.9 KiB
Python
94 lines
2.9 KiB
Python
import re
|
|
|
|
def extract_regex_from_js(js_code):
|
|
pattern1 = r'BypassedByBloggerPemula\((.*?),'
|
|
matches1 = re.findall(pattern1, js_code)
|
|
matches1 = [match.strip('/') for match in matches1]
|
|
|
|
pattern2 = r"BloggerPemula\('([^']+)',"
|
|
matches2 = re.findall(pattern2, js_code)
|
|
#matches2 = ['/' + s + '/' for s in matches2]
|
|
|
|
pattern3 = r"RemoveBp\('([^']+)',"
|
|
matches3 = re.findall(pattern3, js_code)
|
|
|
|
pattern4 = r'case \'(.*?)\':'
|
|
matches4 = re.findall(pattern4, js_code)
|
|
|
|
pattern5 = r"h\.href\.includes\('(.*?)'\)"
|
|
matches5 = re.findall(pattern5, js_code)
|
|
|
|
return matches1+matches2+matches3+matches4+matches5
|
|
|
|
def regex_to_include_line(regex):
|
|
#regex = regex.strip("/")
|
|
|
|
#Use @include for more complex regex
|
|
if any(char in regex for char in ['|', '(', ')', '*']):
|
|
regex = '(' + regex + ')'
|
|
include_line = "// @include /^(https?:\/\/)(.+)?" + regex + "(\/.*)/"
|
|
include_line = include_line.replace( "\.*)(\/.*)/", "\.*)/" ) #clean excess in the regex
|
|
|
|
#Use @match for simpler regex
|
|
else:
|
|
include_line = '// @match *://*.' + regex + '/*'
|
|
|
|
return include_line
|
|
|
|
def generate_include_lines(regex_list):
|
|
include_lines = []
|
|
for regex in regex_list:
|
|
include_line = regex_to_include_line(regex)
|
|
include_lines.append(include_line)
|
|
|
|
#Manual additions of lines
|
|
include_lines.append('// @match *://*/recaptcha/api2/*')
|
|
|
|
return include_lines
|
|
|
|
def write_to_file(filename, lines):
|
|
with open(filename, 'w') as file:
|
|
for line in lines:
|
|
file.write(line + '\n')
|
|
print(f"OK: Generated {filename}")
|
|
|
|
def compile_and_print(regex_strings):
|
|
#for regex_string in regex_strings: print(regex_string)
|
|
write_to_file('supported_sites.txt', regex_strings)
|
|
|
|
include_lines = generate_include_lines(regex_strings)
|
|
print(f"OK: Generated {len(include_lines)} include lines.")
|
|
|
|
#for line in include_lines: print(line)
|
|
write_to_file('includes.txt', include_lines)
|
|
|
|
def remove_strings_containing_word(word, string_list):
|
|
return [s for s in string_list if word not in s]
|
|
|
|
def filter_strings(input_list):
|
|
filtered_list = [string for string in input_list if "." in string and len(string) >= 4]
|
|
filtered_list = remove_strings_containing_word("google", filtered_list)
|
|
return filtered_list
|
|
|
|
|
|
def main():
|
|
file_path = 'untouched_Bypass_All_Shortlinks.user.js'
|
|
|
|
try:
|
|
with open(file_path, 'r', encoding='utf-8') as file:
|
|
js_code = file.read()
|
|
|
|
regex_strings = extract_regex_from_js(js_code)
|
|
regex_strings = filter_strings(regex_strings)
|
|
|
|
#Manual additions
|
|
regex_strings.append('shrinkme.us')
|
|
|
|
compile_and_print(regex_strings)
|
|
except FileNotFoundError:
|
|
print(f"Error: File '{file_path}' not found.")
|
|
except Exception as e:
|
|
print(f"An error occurred: {e}")
|
|
|
|
if __name__ == "__main__":
|
|
main()
|